Try these examples on the Demo GraphQL Playground
Simple Hits#
Graphql-java: The alpha and omega of any project, the GraphQL Java implementation itself. Graphql-java-tools: The library that allows you to use the GraphQL schema language to build your GraphQL schema. It's inspired by Apollo's graphql-tools, it parses the given GraphQL schema and allows you to bring your own object to fill in the implementations. Creates a GraphQLSchema object from GraphQL schema language. The schema will use default resolvers. For more detail on the GraphQL schema language, see the schema language docs or this schema language cheat sheet. Try these examples on the Demo GraphQL Playground. Used for facet interactions such as for search or for when displaying more facet options.
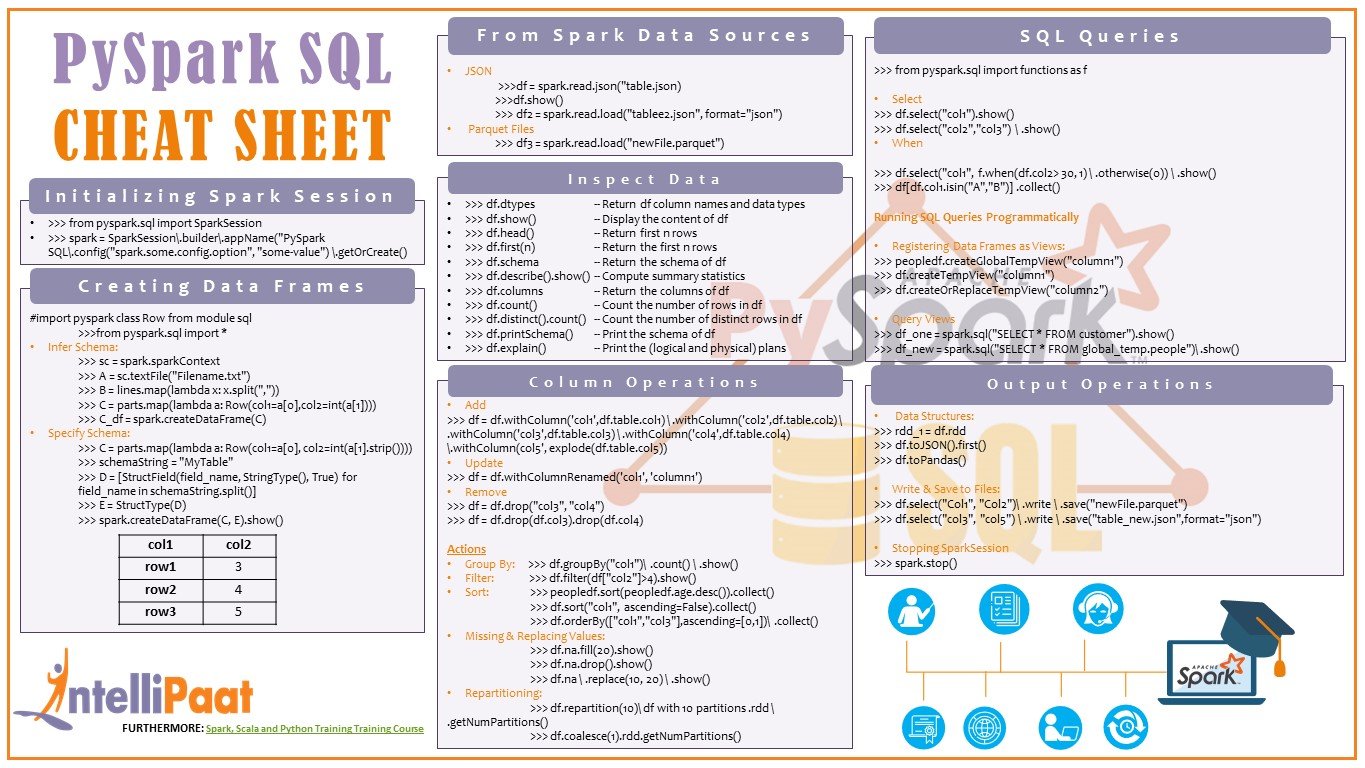
Hit Querying#
Hit Filtering#
Facet list#
Single Facet#
Used for facet interactions such as for search or for when displaying more facet options.
Pagination#
Sorting Results#
Filter Summary#
Graphql Schema Query
The graphql/utilities
module contains common useful computations to use withthe GraphQL language and type objects. You can import either from the graphql/utilities
module, or from the root graphql
module. For example:
Overview#
Introspection
Schema Language
Visitors
Value Validation
Introspection#
introspectionQuery#
A GraphQL query that queries a server's introspection system for enoughinformation to reproduce that server's type system.
buildClientSchema#
Build a GraphQLSchema for use by client tools.
Given the result of a client running the introspection query, creates andreturns a GraphQLSchema instance which can be then used with all GraphQL.jstools, but cannot be used to execute a query, as introspection does notrepresent the 'resolver', 'parse' or 'serialize' functions or any otherserver-internal mechanisms.
Schema Representation#
buildSchema#
Creates a GraphQLSchema object from GraphQL schema language. The schema will use default resolvers. For more detail on the GraphQL schema language, see the schema language docs or this schema language cheat sheet.
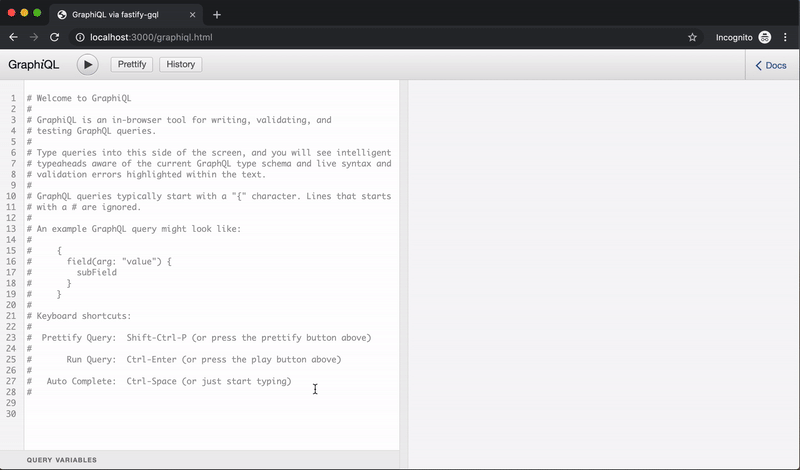
printSchema#
Prints the provided schema in the Schema Language format.
printIntrospectionSchema#
Prints the built-in introspection schema in the Schema Language format. Pages for mac icon.
buildASTSchema#
This takes the ast of a schema document produced by parseSchemaIntoAST
ingraphql/language/schema
and constructs a GraphQLSchema instance which can bethen used with all GraphQL.js tools, but cannot be used to execute a query, asintrospection does not represent the 'resolver', 'parse' or 'serialize'functions or any other server-internal mechanisms.
typeFromAST#
Given the name of a Type as it appears in a GraphQL AST and a Schema, return thecorresponding GraphQLType from that schema.
Graphql Schema Cheat Sheet Example
astFromValue#
Produces a GraphQL Input Value AST given a JavaScript value.
Optionally, a GraphQL type may be provided, which will be used todisambiguate between value primitives.
Visitors#
TypeInfo#
TypeInfo is a utility class which, given a GraphQL schema, can keep trackof the current field and type definitions at any point in a GraphQL documentAST during a recursive descent by calling enter(node)
and leave(node)
.
Graphql Schema Cheat Sheet Excel
Value Validation#
isValidJSValue#
Given a JavaScript value and a GraphQL type, determine if the value will beaccepted for that type. Linux for ppc mac os. This is primarily useful for validating theruntime values of query variables. Httrack for mac.
isValidLiteralValue#
Utility for validators which determines if a value literal AST is valid givenan input type.
Create Graphql Schema
Note that this only validates literal values, variables are assumed toprovide values of the correct type.
